OCR Review
Description
The Review screen provides the user a chance to review (and rectify, if necessary) the information collected through the OCR module. This review screen automatically pre-fills the OCR extracted details. It's a single-page screen that consists of two main sections: Personal details including name, DOB and address, and Document details including ID number.
Default Review screen
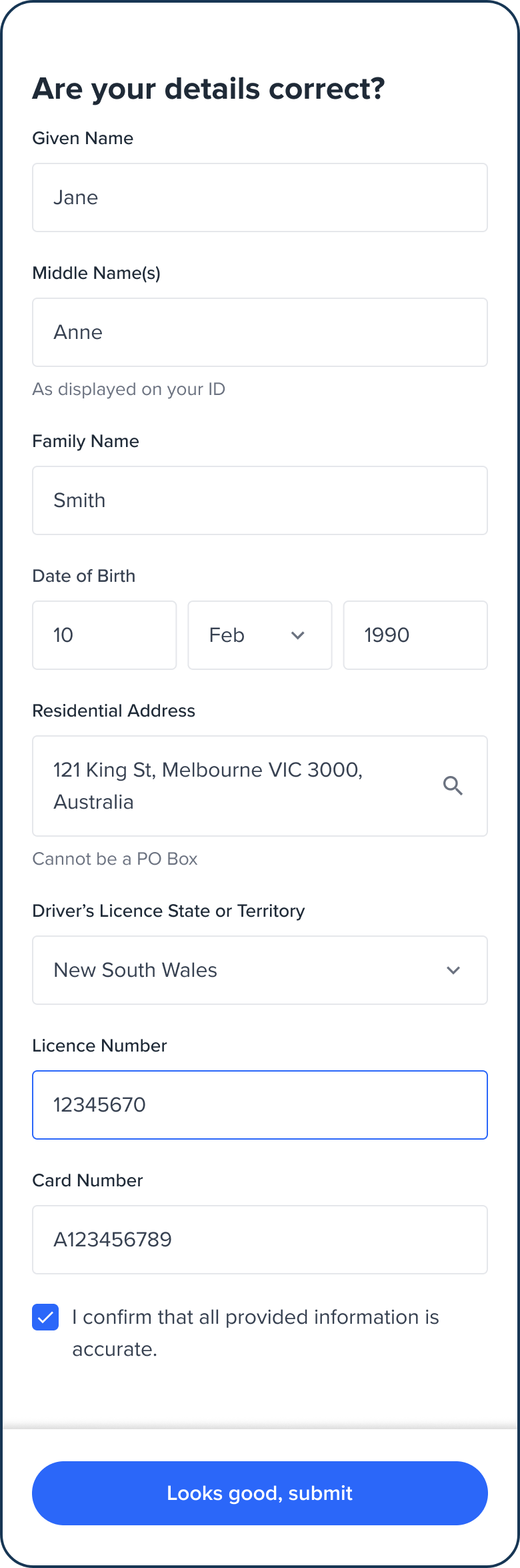
Review screen.
Default Screen
If you render the Review form without any configuration, it renders the default screen as shown above, and it will automatically show relevant ID fields depending on the extracted document type (Driver's Licence, Passport or Medicare).
const form_review = oneSdk.component("form", { name: "REVIEW", type: "ocr", });
If you need to customise the default screen, please use the following section.
Configuration
The configuration of the review screen includes three main items: General, Personal, and Documents.
Both Personal and Document sections follow a similar pattern for configuration and customisation which is explained below.
Field configuration
We have a generic config structure for all the input fields across Personal and Document details. We have several pre-defined fields described in the following sections and if you want to configure and customise them, you need to specify the associated
name
,fieldType
anddataType
.Also note, you don't need to pass all the config attributes, only pass the ones you want to customise.
To learn more about field object, please visit Internationalisation.
1- General
Attribute | Description | Usage |
---|---|---|
name | The name of the screen you want to load | name: REVIEW |
type | The type of the flow to be used. In this use case, it needs to be "ocr" | type:"ocr" |
title | Title of the page. - label: the title text - style: the title style | title: {label: "<Your_TITLE>", style: {'ff-title': {}}} |
style | The global container for the whole page | style ff-form-wrapper |
2- Personal
The personal section contains a set of fields for personal details, such as name and dob, that can be customised and configured with the following pattern:
- givenName
- middleName
- familyName
- dateOfBirth
- address
personal: {
countries:{
default:{
default:{
fields:[
{
fieldType: 'input',
dataType: 'text',
label: `Given Name`,
name: 'givenName',
rules: {
required: {
value: true,
message: 'Please enter your given name',
},
pattern: {...},
}
},
{
fieldType: 'input',
dataType: 'text',
label: `Middle Name(s)`,
name: 'middleName',
...
},
...
]
}
}
}
}
Name
There are three input fields for name, including given name, middle name, and family name, that you can customise the label and validation rules for them, and even remove/show them.
Attribute | Description/ Note |
---|---|
fieldType | For name fields, has to be set to 'input' |
dataType | For name fields, has to be set to 'text' |
label | The label to be displayed for the field |
style | |
name | The name of the field that has been pre-defined in our platform. For the name field, it has to be one of the following:'givenName' 'middleName ''familyName' |
hide | true/false To remove or show the field. If you remove the field, it doesn't get displayed and it won't be submitted to the platform. |
helperText | The text to be displayed below the field to guide users. For example: 'As displayed on your ID' |
rules: | |
required | Specifies whether the field is mandatory or not - value: true/false - message: error message if user doesn't enter name. Example: 'Please enter your given name' |
pattern | regex validation pattern - value: regex value to set validation rules. Example: ^[a-zA-Z]+(\\s+[a-zA-Z]+)*$ - message: error message if user enters invalid. Example: 'Please enter alphabetic characters only' |
DOB
Date input field, including DOB, has a special configuration for calendar which will allow you to configure the type and locale.
Attribute | Description/ Note |
---|---|
fieldType | For DOB field, has to be set to 'date' |
dataType | For DOB field, has to be set to 'text' |
label | The label to be displayed for the field |
style | |
name | The name of the field that has been pre-defined in our platform. For the DOB field, it has to be:'dateOfBirth' |
calendarConfig: | |
type | The type of the calendar:"gregory" : Gregorian(More calendars will be coming soon) |
locale | The locale for the calendar:"en" : English(More locale will be coming come soon) |
day | Specify whether the day of birth is mandatory or not - required : true/false |
month | Specify whether the month of birth is mandatory or not - required: true/false |
year | Specify whether year of birth is mandatory or not - required: true/false |
Address
The Address field by default is using auto search functionality for a better user experience. However, if there's no result found through the search, there's an option for manual address inputs.
Google API Key
For the auto-search address to work, you need to provide your own Google API Key (Google Map). You can do this when you initialise OneSDK:
const oneSdk = await OneSdk({ session: sessionObjectFromBackend, mode: "development", recipe: { form: { provider: { name: 'react', googleApiKey: "<YOUR_API_KEY>>" }, } } });
If you don't provide a google API Key, auto-search cannot find results, and there will be a fallback in the UI for manual input.
Attribute | Description/ Note |
---|---|
fieldType | For name fields, has to be set to 'address' |
dataType | 'current_addr' 'previous_addr' |
label | The label to be displayed for the field |
style | |
name | The name of the field that has been pre-defined in our platform. For the address field, it has to be:'address.fullAddress' |
hide | true/false To remove or show the field. If you remove the field, it doesn't get displayed and it won't be submitted to the platform. |
helperText | The text to be displayed below the field to guide users. For example: 'Cannot be a PO Box' |
manualFieldConfig | Explained below |
manualFieldConfig:
Manual address config consists of a set of separate input fields.
manualFieldConfig: [
{
fieldType: 'select',
dataType: 'text',
hide: false,
label: 'Country',
name: 'address.country',
options: [{label: , value:},{}],
rules: {
required: {
value: true,
message: `Please select your country`,
},
pattern: {
...,
},
},
},
{
fieldType: 'input',
dataType: 'text',
hide: false,
label: 'Unit Number (optional)',
name: 'address.unitNumber',
rules: {
pattern: {
...,
},
},
},
{
fieldType: 'input',
dataType: 'text',
hide: false,
label: 'Street Address',
name: 'address.streetAddress',
rules: {
required: {
value: true,
message: 'Please enter your street address',
},
pattern: {
...,
},
},
},
{
fieldType: 'input',
dataType: 'text',
hide: false,
label: 'Suburb / Town',
name: 'address.town',
rules: {
required: {
value: true,
message: 'Please enter your suburb/town.',
},
pattern: {
...,
},
},
},
{
fieldType: 'select',
dataType: 'text',
hide: false,
label: 'State',
name: 'address.state',
placeholder: 'Select Your State',
options: [{label: , value:},{}],
rules: {
required: {
value: true,
message: 'Please enter your state',
},
pattern: {
...,
},
},
},
{
fieldType: 'input',
dataType: 'text',
hide: false,
label: 'Postcode',
name: 'address.postalCode',
rules: {
required: {
value: true,
message: 'Please enter your postal code',
},
pattern: {
...,
},
},
},
],
Each manual address field follows the same data field structure, for the following field names:
- country
- unitNumber
- postcode
- state
- streetNumber
- streetName
- town
Attribute | Description/ Note |
---|---|
label | label of the field |
name | It has to be one of the following items, based on the field name:'address.country' 'address.unitNumber' 'address.postalCode' 'address.state' 'address.streetNumber' 'address.streetName' 'address.town' |
fieldType | 'select' for country and state'input' for the rest |
dataType | 'text' |
options | List of items to be displayed for a drop-down menu. Only applicable to country and state An array of [{label: "", value: ""}, {label: "", value: ""}] |
rules: | |
required | Specifies whether the field is mandatory or not - value: true/false - message: error message if user doesn't provide any input. Example: 'Please enter your suburb/ town' |
pattern | regex validation pattern - value: regex value to set validation rules. Example: ^[a-zA-Z]+(\\s+[a-zA-Z]+)*$ - message: error message if user enters invalid. Example: 'Please enter alphabetic characters only' |
3- Documents
Document fields can be configured and customised in a similar way. As each document type has a different set of fields, you need to specify an array of fields for each document type, following the below pattern. In the array, only specify the fields you need to configure/customise and skip the rest.
documents: [
{
type: 'DRIVERS_LICENCE',
countries: {
default: {
default: {
fields: [
{
fieldType: 'select',
hide: false,
dataType: 'text',
label: `Driver's Licence State or Territory`,
name: 'region',
rules: {
required: {
value: true,
message: `Please enter your driver's licence number`,
},
minLength: {
value: 1,
message: `Licence number must be at least 1 digits`,
},
maxLength: {
value: 15,
message: `Licence number must not exceed 15 characters`,
},
pattern: { ... }
}
...
},
{
fieldType: 'input',
hide: false,
dataType: 'text',
label: 'Licence Number',
name: 'idNumber',
rules: {
...
},
},
{
fieldType: 'input',
hide: false,
dataType: 'text',
label: 'Card Number',
name: 'extraData.document_number',
rules: {
...
},
},
],
},
},
},
},
{
type: 'PASSPORT',
countries: {
default: {
default: {
fields: [
...
]
},
},
},
},
...
],
Each document type by default has a set of certain fields that you can configure and customise, following the below table:
Attribute | Description/ Note |
---|---|
fieldType | For Driver's Licence state: 'select' For Driver's Licence licence number: 'input' For Driver's Licence card number: 'input' For Medicare card colour: 'select' For Medicare card number: 'input' For Medicare position: 'input' For Medicare expiry: 'date' For Passport country: 'input' For Passport number: 'input' |
dataType | 'text' |
label | The label to be displayed for the field |
style | |
name | The name of the field that has been pre-defined in our platform: For Driver's Licence state: 'region' For Driver's Licence licence number: 'idNumber' For Driver's Licence card number: 'extraData.document_number' For Medicare card colour: 'idSubType' For Medicare card number: 'idNumber' For Medicare position: 'extraData.reference' For Medicare expiry: 'idExpiry' For Passport country: 'country' For Passport number: 'idNumber' |
hide | true/false To remove or show the field. If you remove the field, it doesn't get displayed and it won't be submitted to the platform. |
helperText | The text to be displayed below the field to guide users. For example: 'As displayed on your ID' |
placeholder | The text to be displayed inside the field. For example: 'Select DL State or Territory' |
rules: | |
required | specifies whether the field is mandatory - value: true/ false - message: The error message if user doesn't enter name. Example: 'Please enter your given name' |
minLength | minimum length of the field to be validated - value: integer - message: The error message if user enters less than minimum length |
maxLength | maximum length of the field to be validated - value: integer - message: error message if user enters more than maximum length |
pattern | regex validation pattern - value: regex value to set validation rules. Example: ^[a-zA-Z]+(\\s+[a-zA-Z]+)*$ - message: error message if user enters invalid value. Example: 'Please enter alphabetic characters only' |
Internationalisation
The configuration explained above is a generic configuration applied to all countries and states, by default. The OneSDK modular form will also allow you to overwrite the configuration for any specific country to meet international requirements. To learn more, please visit Internationalisation.
Events
Event name | Description | Arguments |
---|---|---|
form:review:loaded | inputInfo: {type:'ocr'} | |
form:review:ready | Upon clicking on the button, this event will be triggered, and you can listen to it to load another screen. | inputInfo: {type:'ocr'} |
form:review:failed | nputInfo: {type:'ocr'}, message?: string |
UI Customisation
Removing the fields
You can customise the review screen to remove the fields, based on your workflow.
As an example, if you want to remove Date of Birth (DOB) and address for Medicare card, or remove address for Passport, you can define different instances of the review screen and load them based on the document type.
Refer to the code below for examples of how to remove certain fields from the review screen:
const form_review1 = oneSdk.component("form", {
name: "REVIEW",
type: "ocr"
});
const form_review2 = oneSdk.component("form", {
name: "REVIEW",
type: "ocr",
personal: {
countries:{
default:{
default:{
fields:[
{
fieldType: 'address',
name: 'address.fullAddress',
hide: true
}
]
}
}
}
}
});
const form_review3 = oneSdk.component("form", {
name: "REVIEW",
type: "ocr",
personal: {
countries:{
default:{
default:{
fields:[
{
fieldType: 'date',
name: 'dateOfBirth',
hide: true
},
{
fieldType: 'address',
name: 'address.fullAddress',
hide: true
}
]
}
}
}
}
});
Multiple form instances
Even if you render 3 different instances of review form, only listen to one of them upon the
form:review:ready
event and mount Biometrics module:form_review1.on("form:review:ready", async () => { biometrics.mount("<YOUR_CONTAINER>"); });
Styling customisation
You can use the following classes to customise the styling for different elements of the page. These CSS classes apply to the common elements across all the screens. If you want to customise a specific page, you can use the above configuration options to overwrite it.
Item | CSS Class |
---|---|
title | ff-title |
button | ff-button |
Page container | ff-form-wrapper |
Input Fields | ff-form-field |
The following code shows you how to style a specific element on the page, in this example the button's background color will be green, instead of the default blue.
<style>
#<you_div_container> .ff-button {
background-color: green;
}
</style>
Updated 4 months ago